Understanding Object Oriented Programming OOP
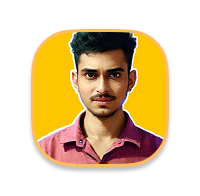
mukesh juadi
Developer passionate about merging technology and creativity in software, games, websites, and more to create engaging experiences.
Learn the fundamentals of Object-Oriented Programming (OOP) with simple examples and key concepts like classes, objects, inheritance, and polymorphism. Explore how OOP improves code reusability, scalability, and efficiency.
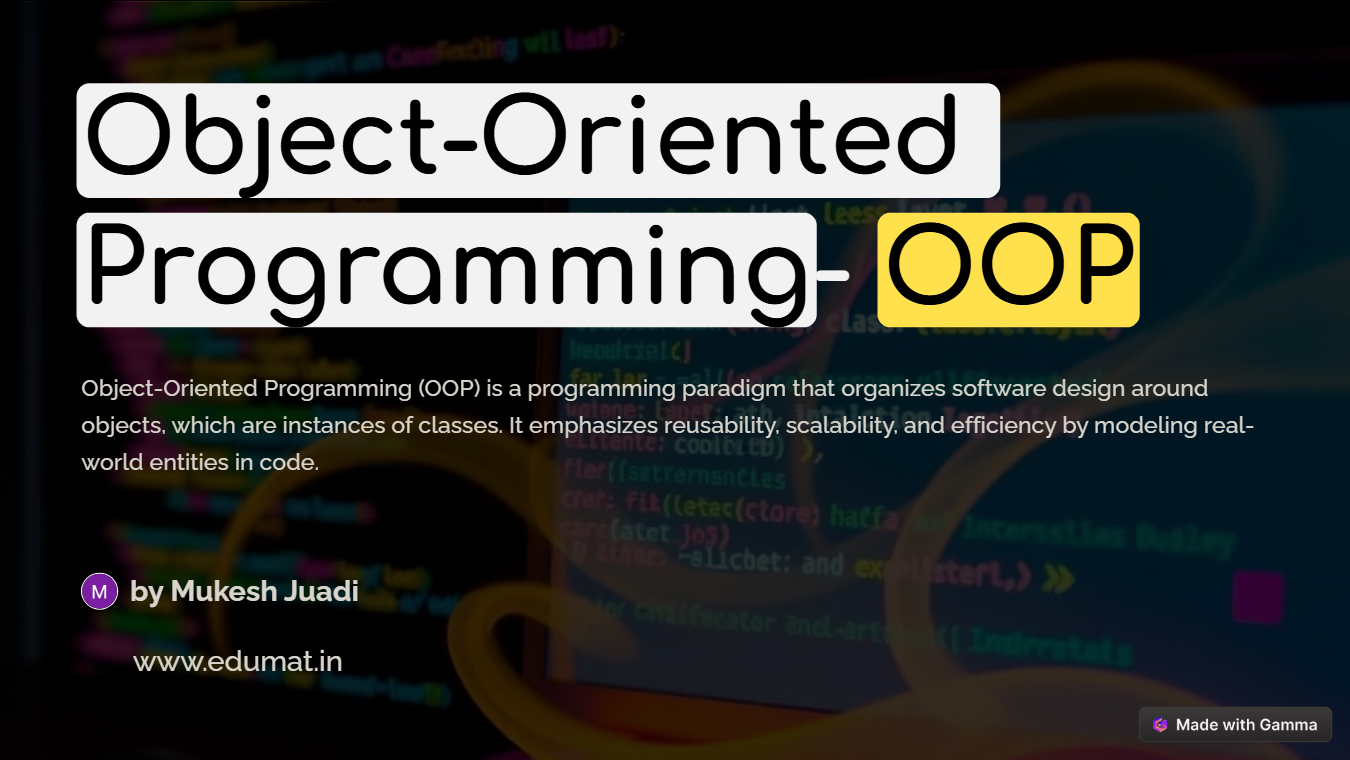
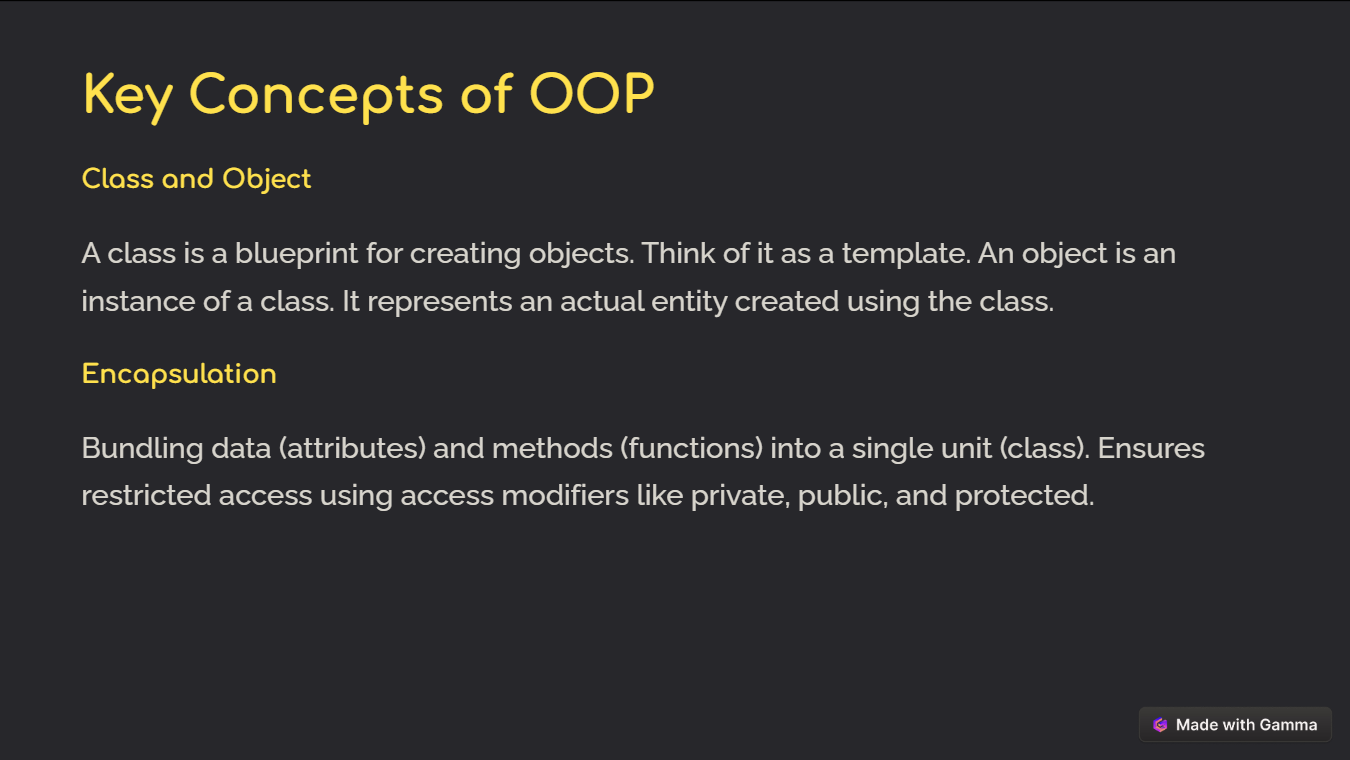
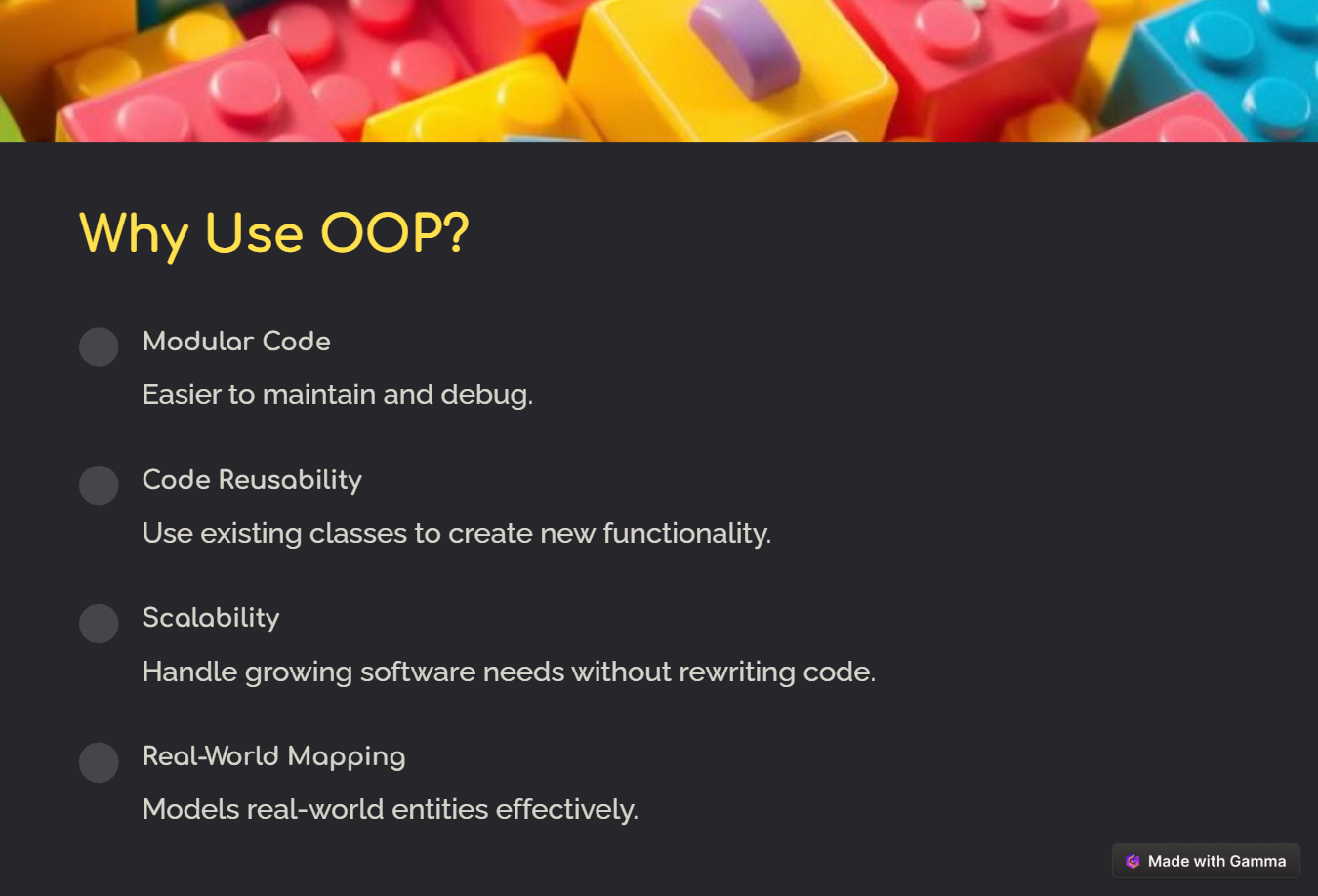
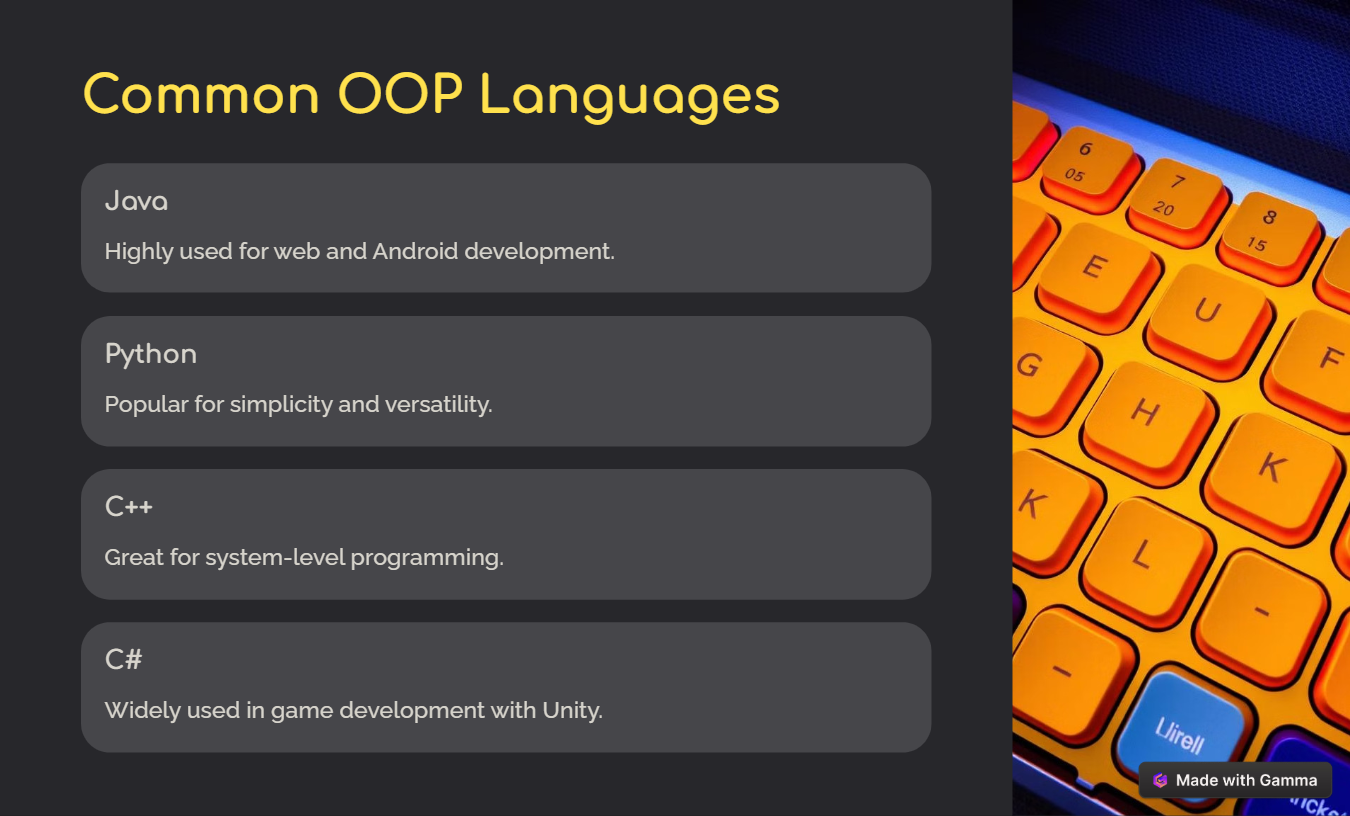
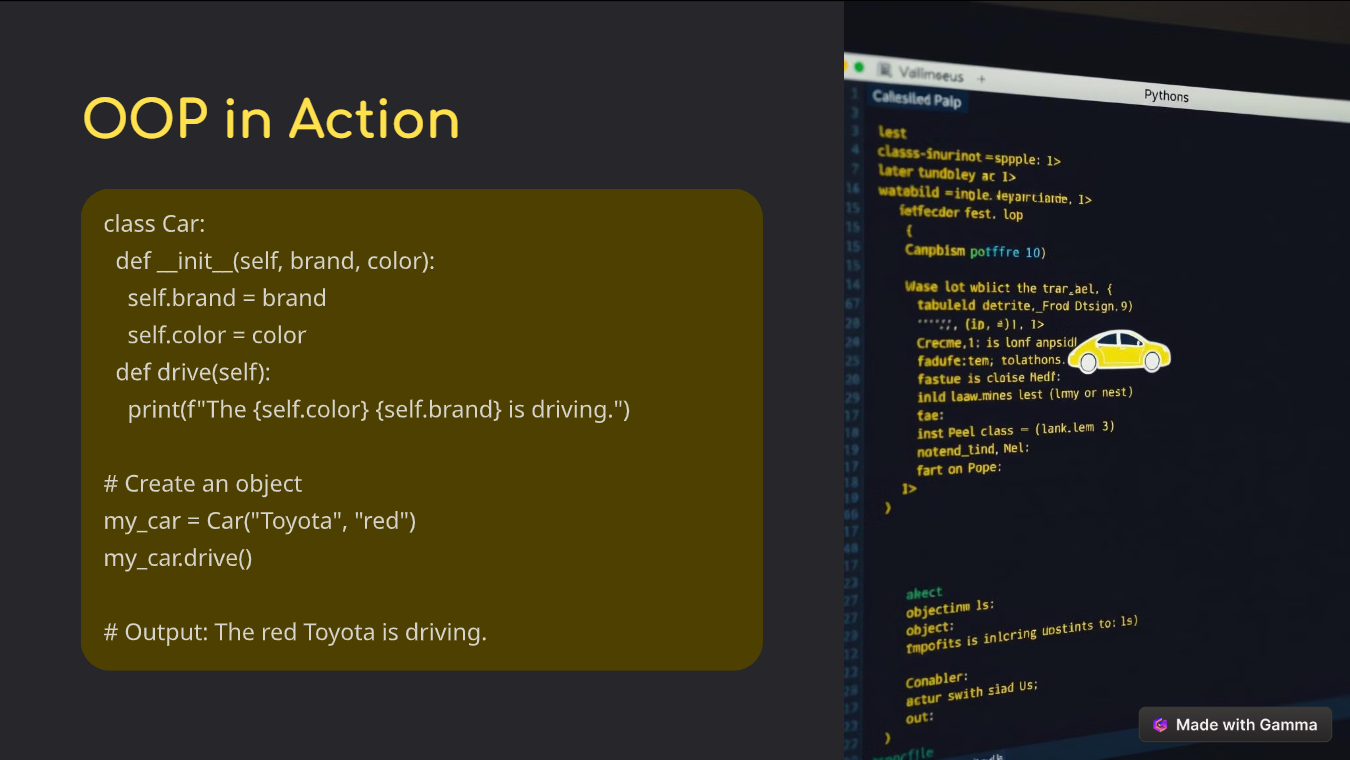
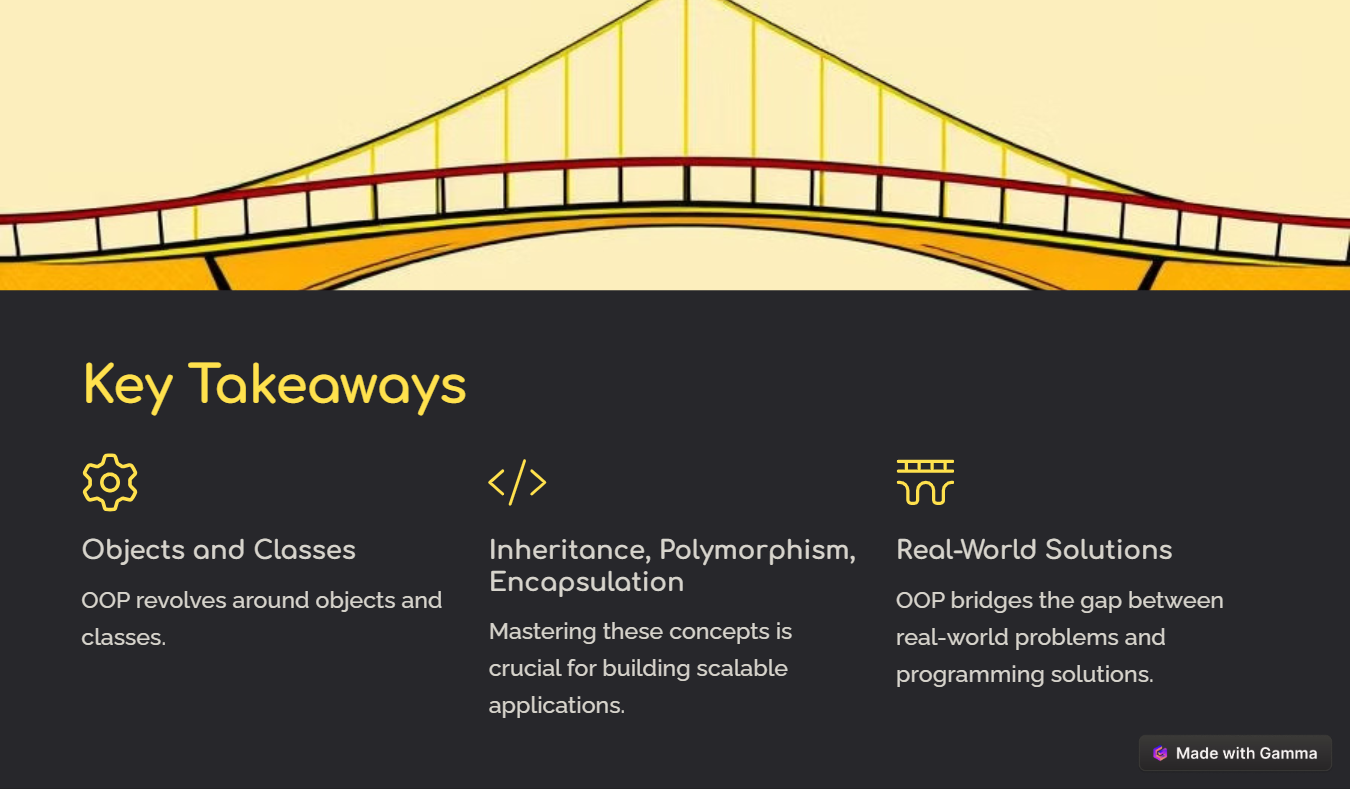
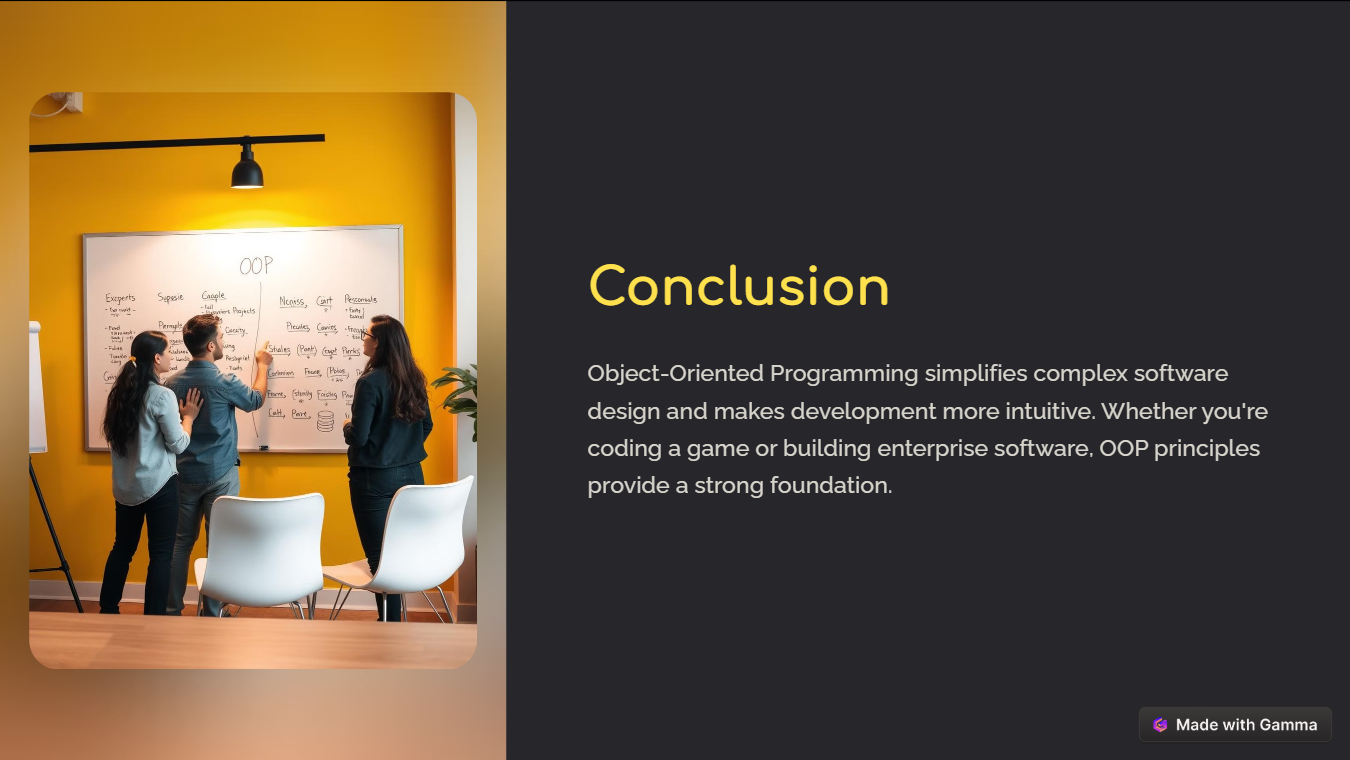
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm that organizes software design around objects, which are instances of classes. It emphasizes reusability, scalability, and efficiency by modeling real-world entities in code.
Key Concepts of OOP
1. Class and Object
- Class: A blueprint for creating objects. Think of it as a template.
- Example: A "Car" class defines properties like color and model and behaviors like drive and stop.
- Object: An instance of a class. It represents an actual entity created using the class.
- Example: A red Toyota is an object of the "Car" class.
2. Encapsulation
- Bundling data (attributes) and methods (functions) into a single unit (class).
- Ensures restricted access using access modifiers like private, public, and protected.
- Example: A class hides its internal state and allows modification only through controlled methods.
3. Inheritance
- A mechanism where one class (child) inherits properties and behaviors from another class (parent).
- Promotes code reusability.
- Example: A "SportsCar" class can inherit from the "Car" class and add unique features like turbo boost.
4. Polymorphism
- Allows methods to perform different tasks based on the object calling them.
- Achieved through method overloading (same name, different parameters) and method overriding (redefining in a subclass).
- Example: A "shape" class has a method "draw"; a circle and square subclass can implement it differently.
5. Abstraction
- Hiding complex implementation details and showing only the essential features.
- Achieved using abstract classes or interfaces.
- Example: A driver operates a car without needing to understand the engine mechanics.
Why Use OOP?
Benefits
- Modular Code: Easier to maintain and debug.
- Code Reusability: Use existing classes to create new functionality.
- Scalability: Handle growing software needs without rewriting code.
- Real-World Mapping: Models real-world entities effectively.
Real-World Examples
- Bank Account System:
- Class: BankAccount (attributes: accountNumber, balance; methods: deposit, withdraw).
- Objects: John’s Account, Mary’s Account.
- E-Commerce Platform:
- Classes: Product, User, Cart.
- Objects: A specific product, a registered user, a shopping cart.
Common OOP Languages
- Java: Highly used for web and Android development.
- Python: Popular for simplicity and versatility.
- C++: Great for system-level programming.
- C#: Widely used in game development with Unity.